DisplayMetrics DisplayMetrics 구조체에서 디스플레이에 대한 정보를 얻을 수 있다.
Android는 직접 픽셀 매핑을 사용하지 않지만 소수의 Density Independent Pixel 값을 사용하여 실제 화면 크기에 맞게 조정한다.
| 방법1. 밀도 상수로 구분
DisplayMetrics metrics = getResources().getDisplayMetrics();
int density = metrics.densityDpi
// DENSITY_xxx 상수 (120, 160, 213, 240, 320, 480 또는 640 dpi) 중 하나
** 실제lcd 픽셀 밀도가 필요한 경우
수평 및 수직 밀도 각각에 대해 metrics.xdpi 및 metrics.ydpi 속성에서 가져올 수 있다.
DisplayMetrics dm = new DisplayMetrics();
getWindowManager().getDefaultDisplay().getMetrics(dm);
// will either be DENSITY_LOW, DENSITY_MEDIUM or DENSITY_HIGH
int dpiClassification = dm.densityDpi;
// these will return the actual dpi horizontally and vertically
float xDpi = dm.xdpi;
float yDpi = dm.ydpi;
| 방법2. 상수값으로 구분
//방법 2 상수
getResources().getDisplayMetrics().density;
/*
0.75 - ldpi
1.0 - mdpi
1.5 - hdpi
2.0 - xhdpi
3.0 - xxhdpi
4.0 - xxxhdpi
*/
// 4이전의 API 수준을 대상으로 지정하는 경우 : metrics.density = 참조밀도(160dpi)의 소수점 배율 인수
int densityDpi = (int)(metrics.density * 160f);
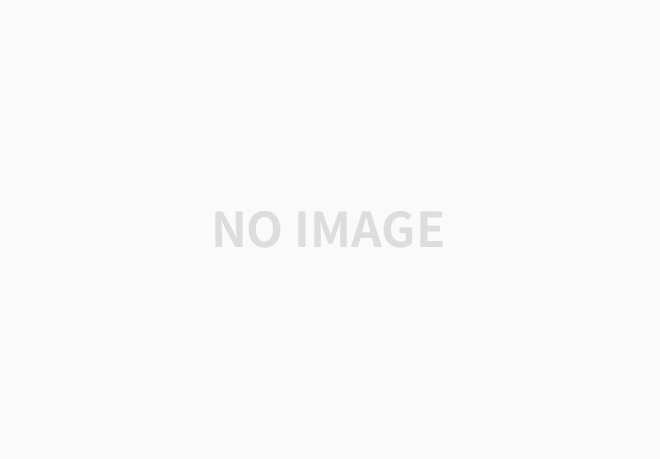
| Sample Code
int densityDpi = getResources().getDisplayMetrics().densityDpi;
switch (densityDpi)
{
case DisplayMetrics.DENSITY_LOW:
// LDPI : density == 0.75f
break;
case DisplayMetrics.DENSITY_MEDIUM:
// MDPI : density >= 1.0f && density < 1.5f
break;
case DisplayMetrics.DENSITY_TV:
case DisplayMetrics.DENSITY_HIGH:
// HDPI : density == 1.5f
break;
case DisplayMetrics.DENSITY_XHIGH:
case DisplayMetrics.DENSITY_280:
// XHDPI : density > 1.5f && density <= 2.0f
break;
case DisplayMetrics.DENSITY_XXHIGH:
case DisplayMetrics.DENSITY_360:
case DisplayMetrics.DENSITY_400:
case DisplayMetrics.DENSITY_420:
// XXHDPI : density > 2.0f && density <= 3.0f
break;
case DisplayMetrics.DENSITY_XXXHIGH:
case DisplayMetrics.DENSITY_560:
// XXXHDPI : density > 4.0f
break;
}
Summary
int | DENSITY_140
Intermediate density for screens that sit between DENSITY_LOW (120dpi) and DENSITY_MEDIUM (160dpi). |
int | DENSITY_180
Intermediate density for screens that sit between DENSITY_MEDIUM (160dpi) and DENSITY_HIGH (240dpi). |
int | DENSITY_200
Intermediate density for screens that sit between DENSITY_MEDIUM (160dpi) and DENSITY_HIGH (240dpi). |
int | DENSITY_220
Intermediate density for screens that sit between DENSITY_MEDIUM (160dpi) and DENSITY_HIGH (240dpi). |
int | DENSITY_260
Intermediate density for screens that sit between DENSITY_HIGH (240dpi) and DENSITY_XHIGH (320dpi). |
int | DENSITY_280
Intermediate density for screens that sit between DENSITY_HIGH (240dpi) and DENSITY_XHIGH (320dpi). |
int | DENSITY_300
Intermediate density for screens that sit between DENSITY_HIGH (240dpi) and DENSITY_XHIGH (320dpi). |
int | DENSITY_340
Intermediate density for screens that sit somewhere between DENSITY_XHIGH (320 dpi) and DENSITY_XXHIGH (480 dpi). |
int | DENSITY_360
Intermediate density for screens that sit somewhere between DENSITY_XHIGH (320 dpi) and DENSITY_XXHIGH (480 dpi). |
int | DENSITY_400
Intermediate density for screens that sit somewhere between DENSITY_XHIGH (320 dpi) and DENSITY_XXHIGH (480 dpi). |
int | DENSITY_420
Intermediate density for screens that sit somewhere between DENSITY_XHIGH (320 dpi) and DENSITY_XXHIGH (480 dpi). |
int | DENSITY_440
Intermediate density for screens that sit somewhere between DENSITY_XHIGH (320 dpi) and DENSITY_XXHIGH (480 dpi). |
int | DENSITY_450
Intermediate density for screens that sit somewhere between DENSITY_XHIGH (320 dpi) and DENSITY_XXHIGH (480 dpi). |
int | DENSITY_560
Intermediate density for screens that sit somewhere between DENSITY_XXHIGH (480 dpi) and DENSITY_XXXHIGH (640 dpi). |
int | DENSITY_600
Intermediate density for screens that sit somewhere between DENSITY_XXHIGH (480 dpi) and DENSITY_XXXHIGH (640 dpi). |
int | DENSITY_DEFAULT
The reference density used throughout the system. |
int | DENSITY_HIGH
Standard quantized DPI for high-density screens. |
int | DENSITY_LOW
Standard quantized DPI for low-density screens. |
int | DENSITY_MEDIUM
Standard quantized DPI for medium-density screens. |
int | DENSITY_TV
This is a secondary density, added for some common screen configurations. |
int | DENSITY_XHIGH
Standard quantized DPI for extra-high-density screens. |
int | DENSITY_XXHIGH
Standard quantized DPI for extra-extra-high-density screens. |
int | DENSITY_XXXHIGH
Standard quantized DPI for extra-extra-extra-high-density screens. |
Fields
public static final int | DENSITY_DEVICE_STABLE
The device's stable density. |
public float | density
The logical density of the display. |
public int | densityDpi
The screen density expressed as dots-per-inch. |
public int | heightPixels
The absolute height of the available display size in pixels. |
public float | scaledDensity
A scaling factor for fonts displayed on the display. |
public int | widthPixels
The absolute width of the available display size in pixels. |
public float | xdpi
The exact physical pixels per inch of the screen in the X dimension. |
public float | ydpi
The exact physical pixels per inch of the screen in the Y dimension. |
Public constructors
DisplayMetrics() |
Public methods
boolean | equals(Object o)
Indicates whether some other object is "equal to" this one. |
boolean | equals(DisplayMetrics other)
Returns true if these display metrics equal the other display metrics. |
int | hashCode()
Returns a hash code value for the object. |
void | setTo(DisplayMetrics o) |
void | setToDefaults() |
String | toString()
Returns a string representation of the object. |
DisplayMetrics | Android 개발자 | Android Developers
developer.android.com
'Mobile > Android' 카테고리의 다른 글
[Android] Android 12 - startScanner java.lang.SecurityException (0) | 2022.02.15 |
---|---|
[Android] 개발자 정책 변경 (6/16일까지 변경 필요) (0) | 2020.05.19 |
[Android] Launching 'app' on Unknown Device. (0) | 2019.10.23 |
[Android] 구글이 알려주는 정기 결제 (Subscription 101) (0) | 2019.10.13 |
[Android] 앱을 Play Console에 업로드 - .aab 파일 (0) | 2019.10.10 |